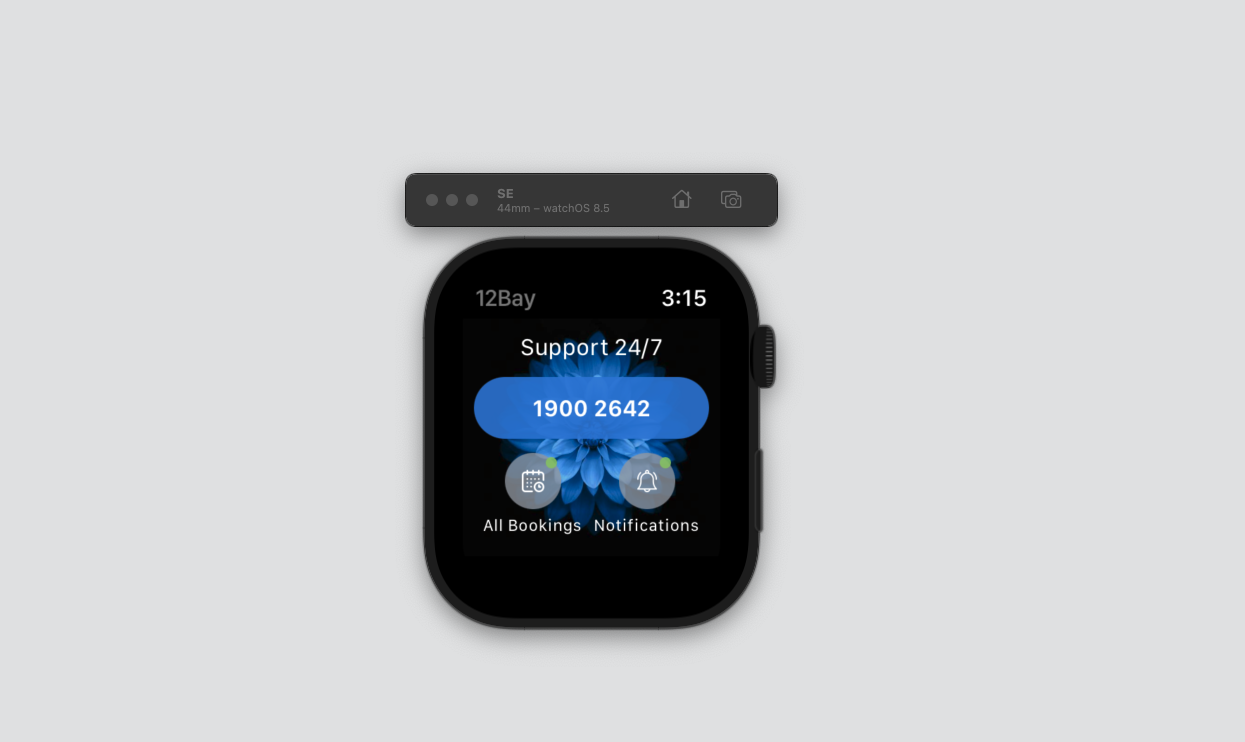
Apple Watch Serial P2 Homescreen Complete
Table of Contents
Abstract
Today, We will complete the homescreen. In this part, we just add background images and events for the elements view. Here are some keys you will get through this post:
- Using
ZStack
to set full background image. - Make calls from the watch application.
This article is part of my Apple Watch by SwiftUI series.
Complete Home screen UI design
Add background images
We will using Zstack
. In SwiftUI
using “ZStack is a view that overlays its children, aligning them in both axes. - apple”
The ZStack
assigns each successive child view a higher z-axis value than the one before it, meaning later children appear “on top” of earlier ones.The following example creates a ZStack of 100 x 100 point Rectangle views filled with one of six colors, offsetting each successive child view by 10 points so they don’t completely overlap:
let colors: [Color] =
[.red, .orange, .yellow, .green, .blue, .purple]
var body: some View {
ZStack {
ForEach(0..<colors.count) {
Rectangle()
.fill(colors[$0])
.frame(width: 100, height: 100)
.offset(x: CGFloat($0) * 10.0,
y: CGFloat($0) * 10.0)
}
}
}
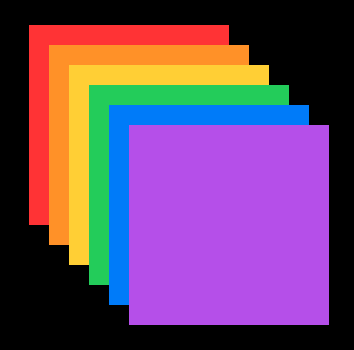
ZStack Alignment (source apple)
Here is the complete HomeView
code after add random background images:
//
// HomeView.swift
// watchOS WatchKit Extension
//
// Created by Tuan Truong Quang on 5/5/22.
// Copyright © 2022 Truong Quang Tuan. All rights reserved.
//
import SwiftUI
struct HomeView: View {
@StateObject fileprivate var service = WatchConnectivityManager()
fileprivate let backgroundImages = ["home-bg", "home-bg1", "home-bg2", "home-bg3", "home-bg4"]
var body: some View {
NavigationView {
ZStack.init {
Image(backgroundImages.randomElement() ?? "home-bg")
.resizable()
.aspectRatio(contentMode: .fill)
VStack(alignment: .center, spacing: 10.0) {
Text("Support 24/7")
Button.init(role: .none, action: {
let phone = "0945822474"
if let telURL = URL(string: "tel:\(phone)") {
let wkExt = WKExtension.shared()
wkExt.openSystemURL(telURL)
}
}, label: {
Text("1900 2642")
.bold()
})
.foregroundColor(.white)
.background(Color.init(hex: 0xE49E26))
.cornerRadius(30.0)
HStack.init(alignment: .center, spacing: 8.0) {
HomeItemView(item: .init(title: "All Bookings", icon: "calendar"))
HomeItemView(item: .init(title: "Notifications", icon: "notification"))
}
}.padding()
}
.alert(item: $service.notificationMessage, content: { info in
Alert(title: Text("WELL DONE\n\(info.messsage)"))
})
}
.navigationTitle("12Bay")
.navigationBarTitleDisplayMode(.inline)
.navigationViewStyle(.stack)
.navigationBarHidden(false)
.navigationBarTitleDisplayMode(.inline)
}
}
struct HomeView_Previews: PreviewProvider {
static let devices:[String] = ["Apple Watch Series 6 - 44mm", "Apple Watch SE - 40mm"]
static var previews: some View {
ForEach(HomeView_Previews.devices, id: \.self) { devicesName in
HomeView()
.previewDevice(PreviewDevice(rawValue: devicesName))
.previewDisplayName(devicesName)
}
}
}
Now, We can build and run the app. This image below run on Apple Watch Series 6 - 44mm
simulator.
Add events
-
Button
touch to make support call centerlet phone = "19002642" if let telURL = URL(string: "tel:\(phone)") { let wkExt = WKExtension.shared() wkExt.openSystemURL(telURL) }
In which,
WKExtension
The centralized point of control and coordination for apps running in watchOS.
shared()
Returns the shared WatchKit extension object.
openSystemURL()
Opens the specified system URL. -
HomeItemView
All Bookings: Push to the my booking screen. -
HomeItemView
Notifications: Push to the my notification screen.
Conclusion
That’s all, we complete the homescreen. In the next post, I will create the my booking screen and using the watch connectivity
to send my order data from iPhone to watch and display it. Happy coding.
Posts in this Series
- Apple Watch Serial Summary
- Apple Watch Serial My Notification
- Apple Watch Serial - My Order Detail Screen
- Apple Watch Serial - My Order Screen
- Apple Watch Serial P2 Homescreen Complete
- Apple Watch Serial P2 Homescreen Connect
- Apple Watch Serial P1 Homescreen
- Let's Idea, How to Design Travel App (12Bay) on WatchOS?
- Apple Watch Series