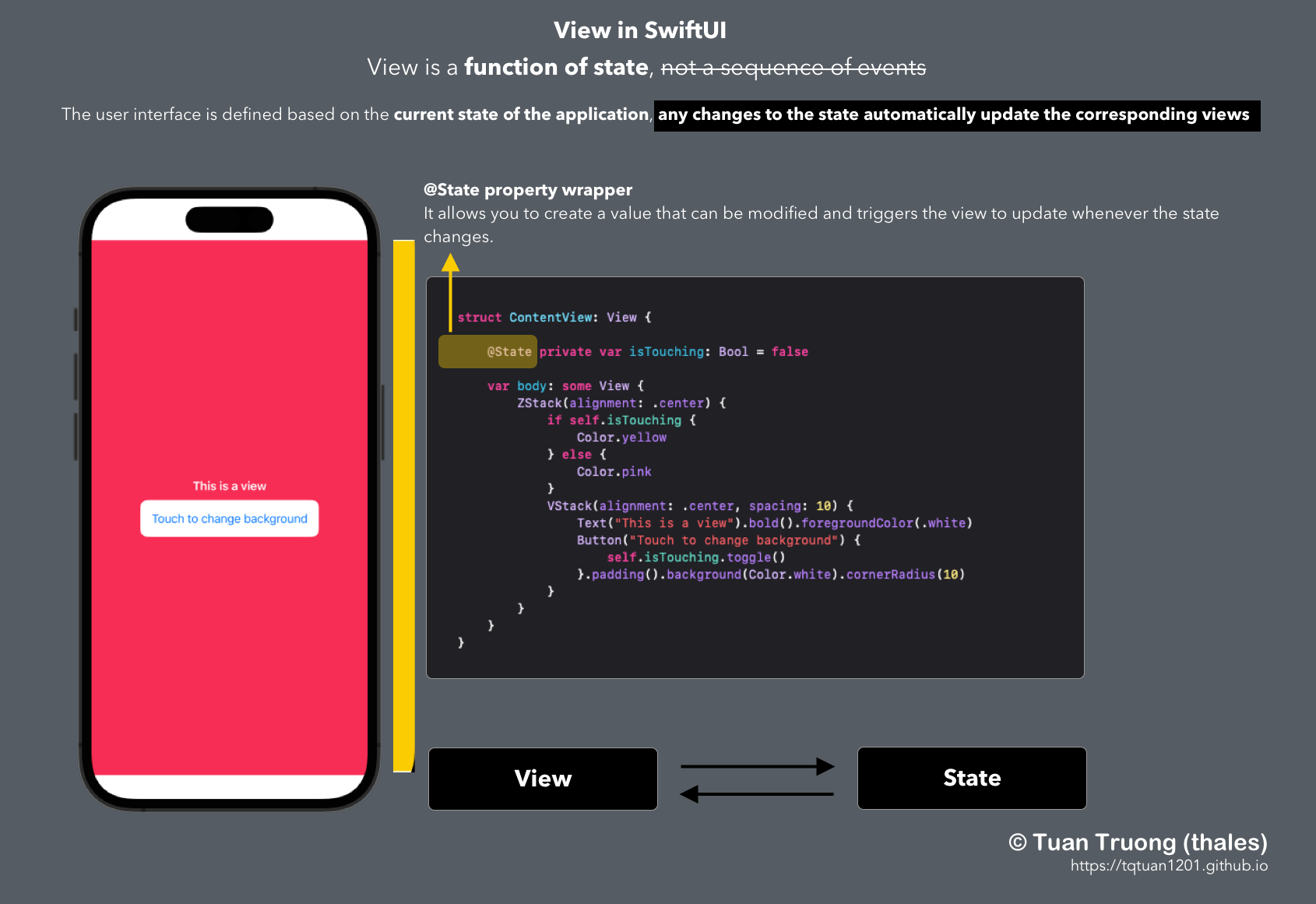
Understand View in SwiftUI
Table of Contents
Abstract
In this article, we will learn about view
in SwiftUI. This article is part of the SwiftUI Series - SwiftUI Series - Updating TTBaseUIKit to support SwiftUI
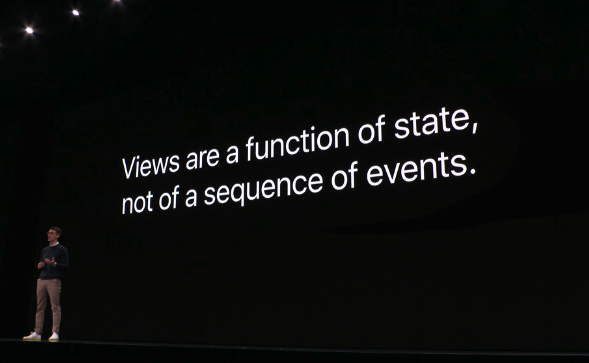
View is a function of state, not a sequence of events - apple
Woaaaa !
SwiftUI
- Apple’s declarative framework for building user interfaces. The user interface is defined based on the current state
of the application, and any changes to the state automatically update the corresponding views.
What is a View?
View
: A type that represents part of your app’s user interface and provides modifiers that you use to configure views. - apple
You create custom views by declaring types that conform to the View
protocol. Implement the required body
computed property to provide the content for your custom view. view-apple-overview
struct MyView: View {
var body: some View {
Text("Hello, World!")
}
}
There are two points we need to note.
Firstly, struct
style
In SwiftUI, the struct
keyword is used to define views because structs in Swift have value semantics. This means that when you create an instance of a struct, it is copied rather than referenced. This property is important in SwiftUI because the framework relies on struct values to manage the state and rendering of views efficiently.
- Value Semantics: Structs in Swift have value semantics, which makes them easier to reason about and avoids common pitfalls associated with shared mutable state. Each instance of a struct is independent, and modifying one instance does not affect other instances.
- Immutable Views: SwiftUI promotes the use of immutable views. When the state of a view changes, SwiftUI creates a new instance of the view with the updated state, rather than modifying the existing view. This approach allows SwiftUI to optimize view updates and perform efficient diffs to update only the necessary parts of the UI.
- Performance: The value semantics of structs in SwiftUI allow for efficient memory management and performance optimizations. SwiftUI can compare the old and new values of a struct to determine what needs to be updated, avoiding unnecessary work and improving performance.
- SwiftUI’s View Protocol: The
View
protocol in SwiftUI is designed to work with value types, such as structs. By conforming to theView
protocol, a struct can define its body as a computed property, allowing SwiftUI to efficiently render and update the view hierarchy.
Secondly, body: some View
the
body
property is a requirement of theView
protocol
The body
property is responsible for describing the structure and hierarchy of a view’s user interface. It can contain multiple views, such as stacks, buttons, images, and more, allowing you to build complex and interactive user interfaces using SwiftUI’s composability and declarative syntax.
Model data
Manage the data that your app uses to drive its interface.
SwiftUI offers a declarative approach
to user interface design. As you compose a hierarchy of views, you also indicate data dependencies for the views. When the data changes, either due to an external event or because of an action that the user performs, SwiftUI automatically updates the affected parts of the interface. As a result, the framework automatically performs most of the work that view controllers traditionally do.
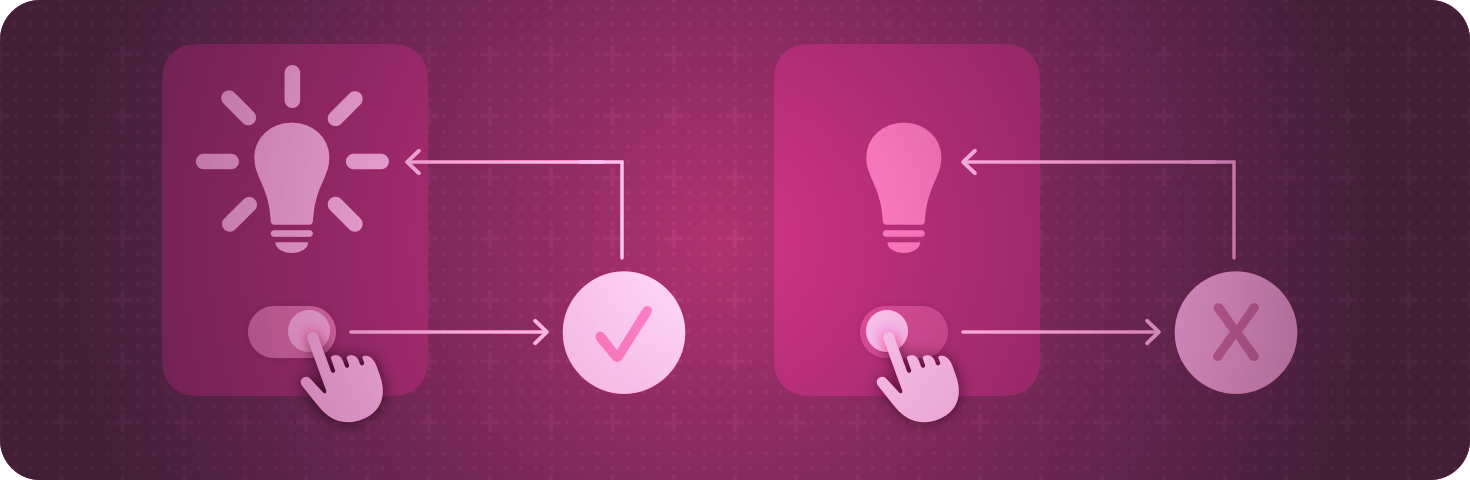
model-data source: apple
The framework provides tools, like state variables and bindings, for connecting your app’s data to the user interface. These tools help you maintain a single source of truth for every piece of data in your app, in part by reducing the amount of glue logic you write. Select the tool that best suits the task you need to perform:
- Manage transient UI state
locally
within a view by wrapping value types asState
properties. Share
a reference to a source of truth, like local state, using theBinding
property wrapper.- Connect to and observe reference model data in
views
by applying theObservable()
macro to the model data type. Instantiate an observable model data type directly in a view using aState
property. Share the observable model data with other views in the hierarchy without passing a reference using theEnvironment
property wrapper.
Summary
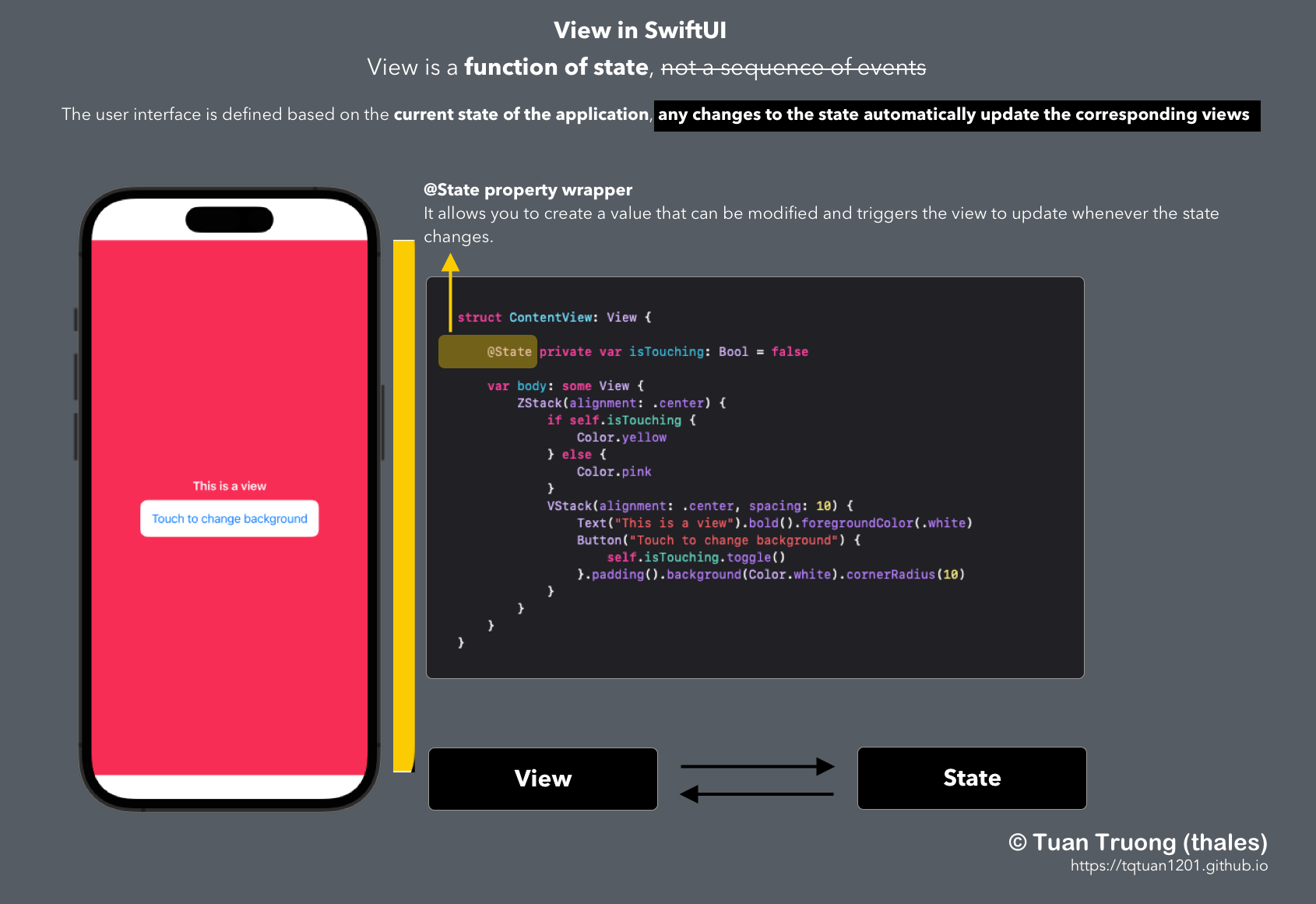
What is a View?
Let’s Code
Hello, World!
The
Text
view is used to display static or dynamically generated text content.
struct ContentView: View {
var body: some View {
Text("Hello, World!")
}
}
Change background color
struct ContentView: View {
@State private var isTouching: Bool = false
var body: some View {
ZStack(alignment: .bottom) {
if self.isTouching {
Color.yellow
} else {
Color.pink
}
VStack(alignment: .center, spacing: 10) {
Text("This is a view").bold().foregroundColor(.white)
Button("Touch to change background") {
self.isTouching.toggle()
}.padding().background(Color.white).cornerRadius(10)
}.padding(.bottom, 80)
}
}
}
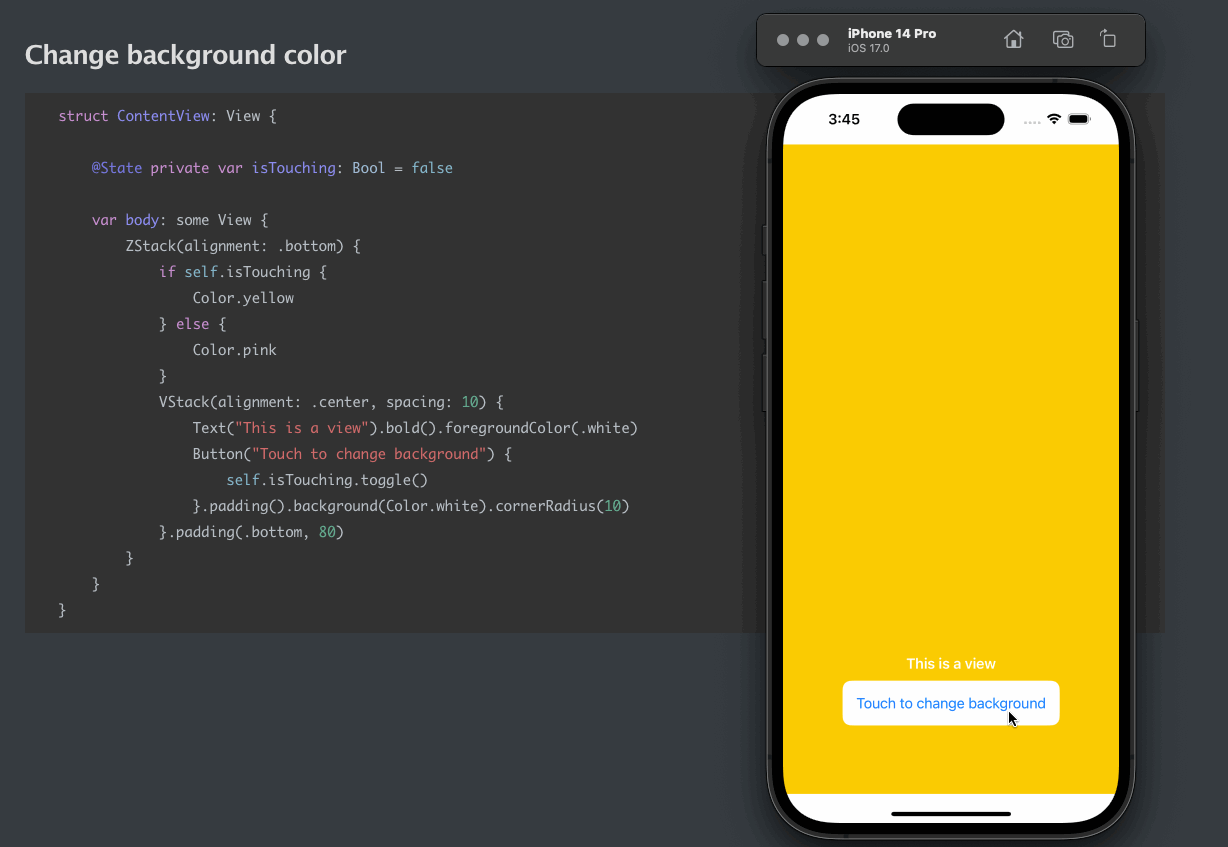
Let’s play!
Conclusion
Everything is a View
Views
are the fundamental building blocks in SwiftUI, responsible for defining the visual elements and interactions within an application. From displaying text and images to creating complex layouts and custom controls, views provide the necessary tools to build dynamic and engaging user interfaces. Have fun and thanks for reading!
Posts in this Series
- TTBaseUIKit Has Been Integrated With SwiftUI Since Version 2.1.0
- Rebuiding Train Booking Feature by SwiftUI in 12Bay Application - Design
- What Is the Spacer and How Do We Use It in SwiftUI
- 12Bay Integrated SwiftUI. With 12Bay, No StoryBoard, No XIB Files, No Cocoapods, ...
- Understand View in SwiftUI
- Understand Safe Area in SwiftUI
- WWDC23 From the Perspective of an IOS Developer
- SwiftUI Series - Updating TTBaseUIKit to Support SwiftUI
If you enjoy reading my articles and find them helpful, please support me. Your support will encourage me to create and share more content with you ^^
By me a coffee